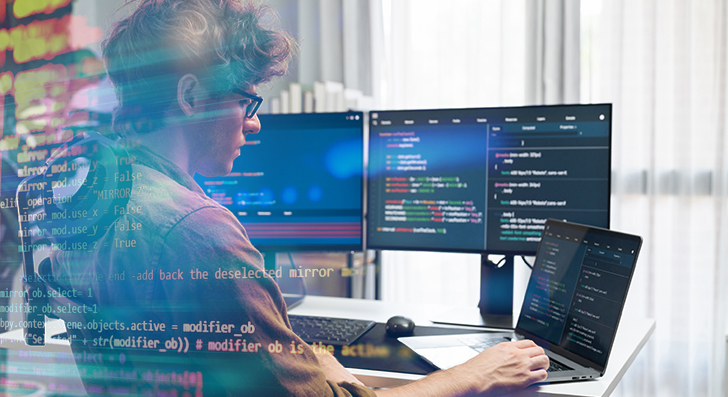
Scalability suggests your application can handle advancement—additional consumers, much more facts, plus much more targeted traffic—with no breaking. As being a developer, building with scalability in your mind saves time and strain later on. Listed here’s a transparent and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on afterwards—it should be part of the plan from the start. Many apps fail whenever they grow speedy due to the fact the first design and style can’t tackle the extra load. For a developer, you have to Assume early about how your process will behave under pressure.
Start off by designing your architecture for being adaptable. Steer clear of monolithic codebases the place everything is tightly linked. As a substitute, use modular layout or microservices. These styles break your app into scaled-down, unbiased components. Every single module or company can scale on its own without having influencing the whole program.
Also, contemplate your databases from working day 1. Will it want to manage one million consumers or maybe 100? Pick the ideal type—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, Even when you don’t need to have them yet.
An additional crucial place is to stay away from hardcoding assumptions. Don’t write code that only functions beneath recent ailments. Take into consideration what would come about If the consumer foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that support scaling, like concept queues or celebration-pushed units. These assist your application deal with a lot more requests with no finding overloaded.
Any time you Create with scalability in your mind, you're not just getting ready for success—you're reducing future problems. A perfectly-prepared system is less complicated to take care of, adapt, and increase. It’s far better to organize early than to rebuild later.
Use the proper Database
Choosing the ideal databases is often a critical Section of creating scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can sluggish you down as well as result in failures as your app grows.
Start out by comprehension your information. Can it be hugely structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and consistency. In addition they assistance scaling procedures like read through replicas, indexing, and partitioning to handle additional website traffic and information.
If the info is a lot more flexible—like consumer activity logs, merchandise catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your go through and produce patterns. Do you think you're performing numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a hefty publish load? Take a look at databases that may take care of superior publish throughput, or simply event-based mostly facts storage units like Apache Kafka (for temporary info streams).
It’s also clever to Imagine ahead. You may not require advanced scaling attributes now, but selecting a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info based upon your obtain styles. And always monitor database overall performance as you develop.
In brief, the proper database depends upon your app’s construction, pace requirements, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save loads of issues later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each little delay provides up. Inadequately composed code or unoptimized queries can slow down overall performance and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Start off by creating clean, very simple code. Prevent repeating logic and remove something unnecessary. Don’t pick the most advanced Resolution if a simple a person will work. Keep your capabilities limited, focused, and straightforward to test. Use profiling tools to search out bottlenecks—areas in which your code takes far too extended to operate or employs an excessive amount of memory.
Future, examine your databases queries. These typically slow factors down greater than the code itself. Be sure Every question only asks for the info you really have to have. Stay away from Find *, which fetches almost everything, and instead decide on specific fields. Use indexes to speed up lookups. And steer clear of executing a lot of joins, Specifically throughout big tables.
In case you notice the identical details becoming requested many times, use caching. Shop the outcome temporarily making use of applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts here down on overhead and helps make your application more effective.
Remember to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash every time they have to handle 1 million.
In brief, scalable apps are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps assist your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle far more people plus much more targeted visitors. If all the things goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the get the job done, the load balancer routes people to diverse servers depending on availability. This means no one server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this easy to arrange.
Caching is about storing facts briefly so it can be reused promptly. When end users request a similar information and facts yet again—like a product web site or possibly a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static data files close to the consumer.
Caching minimizes databases load, increases pace, and will make your app more successful.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but strong applications. With each other, they assist your application deal with extra customers, keep speedy, and Recuperate from troubles. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application improve very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize setup time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy components or guess future ability. When website traffic boosts, you could increase more resources with just a few clicks or automatically utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also offer solutions like managed databases, storage, load balancing, and security tools. You can focus on creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application works by using several containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the application crashes, it restarts it mechanically.
Containers also ensure it is easy to different portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for effectiveness and reliability.
To put it briefly, making use of cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get better rapidly when complications take place. If you prefer your application to grow with no restrictions, commence applying these resources early. They help save time, reduce chance, and assist you remain centered on setting up, not fixing.
Keep an eye on Everything
Should you don’t watch your software, you won’t know when items go Completely wrong. Monitoring can help the thing is how your app is executing, place challenges early, and make better choices as your app grows. It’s a critical Element of developing scalable techniques.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app as well. Keep watch over how long it will take for consumers to load webpages, how often problems come about, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes earlier mentioned a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, often right before people even detect.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it will cause authentic hurt.
As your app grows, targeted visitors and knowledge boost. Without checking, you’ll skip indications of problems until it’s far too late. But with the correct tools in position, you stay on top of things.
In brief, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small applications need a powerful Basis. By creating thoroughly, optimizing wisely, and utilizing the ideal equipment, you could Construct applications that grow easily devoid of breaking under pressure. Commence compact, Believe massive, and Establish wise.